Pengurutan merupakan proses dasar yang ada dalam algoritma dan stuktur data. Algoritma Bubble Sort adalah pengurutan dengan cara pertukaran data dengan data disebelahnya secara terus menerus sampai dalam satu iterasi tertentu tidak ada lagi perubahan.
Algoritma Bubble Sort ini merupakan proses pengurutan yang secara berangsur-angsur berpindah ke posisi yang tepat.
Di bawah ini merupakan gambaran dari algoritma Bubble Sort dengan array “1,3,7,6,5”.
Proses pertama
(1, 3, 7, 6, 5) menjadi (1, 3, 7, 6, 5)
(1, 3, 7, 6, 5) menjadi (1, 3, 7, 6, 5)
(1, 3, 7, 6, 5) menjadi (1, 3, 6, 7, 5)
(1, 3, 6, 7, 5) menjadi (1, 3, 6, 5, 7)
Proses kedua
(1, 3, 6, 5, 7) menjadi (1, 3, 6, 5, 7)
(1, 3, 6, 5, 7) menjadi (1, 3, 6, 5, 7)
(1, 3, 6, 5, 7) menjadi (1, 3, 5, 6, 7)
(1, 3, 5, 6, 7) menjadi (1, 3, 5, 6, 7)
Algoritma Bubble Sort ini mempunyai kelebihan dan kekurangan, untuk kelebihannya metode ini merupakan metode paling sederhana untuk mengurutkan data. Selain sederhana, algoritma Bubble Sort mudah dipahami. Sementara itu, kekurangannya terletak pada efisiensi.
Bubble Sort ini merupakan metode pengurutan yang tidak efisien karena ketika mengurutkan data yang sangat besar akan sangat lambat prosesnya. Selain itu, jumlah pengulangan akan tetap sama jumlahnya meskipun data sudah cukup terurut.
Program Bubble Sort pada Java
Program 1 Bubble Short Java
public class Program
{
static void bubbleSort(int[ ] lst){
boolean swapped;
int space = lst.length;
//a do-while loop to prevent futher itration after the list is sorted.
do{
swapped=false;
for(int i=0;i<space-1;i++){
if(lst[i+1]<lst[i]){
int first=lst[i];
lst[i]=lst[i+1];
lst[i+1]=first;
swapped = true;
}
}
//displays list after every sort
System.out.print("==> ");
for(int i=0; i<space;i++){
System.out.print(lst[i]+" ");
}
System.out.print("\n");
}while(swapped==true);
//displays the fully bubbleSorted list.
System.out.print("\n\nFinally: ");
for(int each:lst){
System.out.print(each+" ");
}
}
public static void main(String[] args) {
int[] nums ={3,4,1,6,0,2,8};
bubbleSort(nums);
}
}
Hasil :
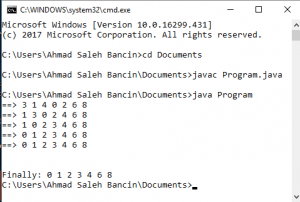
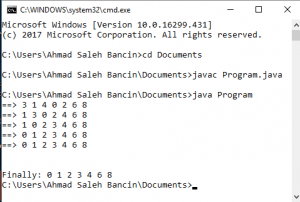
Program 2 Bubble Short Java
import java.util.Arrays;
import java.util.Random;
public class Program2 {
public static void main(String[] args) {
int[] list = new int[10];
// Fill array with numbers
for (int i = 0; i < list.length; i++) {
Random random = new Random();
list[i] = random.nextInt((20 – 1) + 1) + 1;
}
System.out.println("Original: \n" + Arrays.toString(list).replace("[", "").replace("]", "").replace(",", "") + "\n");
for (int j = 0; j < list.length; j++) {
for (int i = 0; i < list.length; i = i + 1) { if (i != list.length – 1) { if (list[i] > list[i + 1]) {
int temp = list[i];
list[i] = list[i + 1];
list[i + 1] = temp;
}
}
}
System.out.println("==> " + Arrays.toString(list).replace("[", "").replace("]", "").replace(",", ""));
}
System.out.println("\nSorted: \n" + Arrays.toString(list).replace("[", "").replace("]", "").replace(",", ""));
}
}
Hasil :
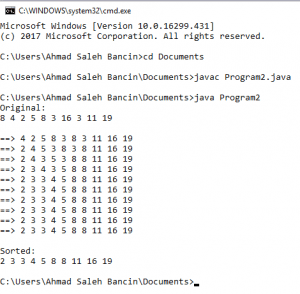
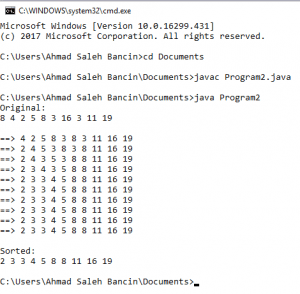
Code 3 Bubble Short Java
public class BubbleSort {
static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
{
// swap arr[j+1] and arr[i]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
// Prints the array
static void printArray(int[] arr) {
int n = arr.length;
for (int i=0; i<n; ++i)
System.out.print(arr[i] + " ");
System.out.println();
}
// Driver method to test above
public static void main(String args[]) {
int [] arr = {1,3,7,6,5};
bubbleSort(arr);
System.out.println("Sorted array:");
printArray(arr);
}
}
Program Bubble Sort pada C++
Program 1
#include <iostream>
#include <cstdlib>
using namespace std;
void bubble_sort(int arr[], int size);
void swap(int& i, int& j);
void present_array(int arr[], int size);
int main()
{
int list[7] = {8, 6, 7, 5, 3, 0, 9};
int list_size = 7;
cout << "List Pre-Swap: ";
present_array(list, list_size);
bubble_sort(list, list_size);
cout << "List Post-Swap: ";
present_array(list, list_size);
return 0;
}
void bubble_sort(int arr[], int size)
{
int i;
int j;
// First "for" loop selects each element.
for (i = 0; i < size; i++)
{
for (j = i + 1; j < size; j++) { if (arr[i] > arr[j])
{
swap(arr[i], arr[j]);
}
}
}
// Second "for" loop swaps while the selected element is greater than its neighbor.
return;
}
void swap(int& i, int& j)
{
int temp;
temp = i;
i = j;
j = temp;
return;
}
void present_array(int arr[], int size)
{
int i;
for (i = 0; i < size; i++)
{
cout << arr[i] << " ";
}
cout << endl;
return;
}
Program 2
#include <iostream>
using namespace std;
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
void bubbleSort(int arr[], int n) {
bool swapped;
for (int i = 0; i < n-1; i++) {
swapped = false;
for (int j = 0; j < n-i-1; j++) { if (arr[j] > arr[j+1]) {
swap(&arr[j], &arr[j+1]);
swapped = true;
}
}
if (swapped == false)
break;
}
}
void printArray(int arr[], int size) {
for (int i =0; i < size; i++)
cout << arr[i] << " ";
}
int main() {
int arr[] = {5, 2, 42, 6, 1, 3, 2};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
printArray(arr, n);
return 0;
}
Code 3
#include <iostream>
using namespace std;
int main()
{
int arr[9] = {1,3,7,6,5,2,10,25,4};
int temp;
for (int i = 0; i < 9; i++)
{
for (int j = 0; j < 9; j++)
{
if (arr[j] > arr[j + 1])
{
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
cout << "Array setelah diurutkan dengan bubble sort:" << endl;
for (int k = 0; k < 9; k++)
{
cout << arr[k] <<" ";
}
return 0;
}
Program Bubble Sort pada C# (C Sharp)
Program 1
using System;
namespace SoloLearn {
class Program {
static void bubbleSort(int[] arr) {
bool swapped;
for(int i = 0; i < arr.Length – 1; i++) {
swapped = false;
for(int j = 0; j < arr.Length – i – 1; j++) { if(arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true;
}
}
if(swapped == false)
break;
}
}
static void printArray(int[] arr) {
for(int i = 0; i < arr.Length; i++) {
Console.Write(arr[i] + " ");
}
}
static void Main(string[] args) {
int [] arr = {5, 2, 42, 6, 1, 3, 2};
E(arr);
printArray(arr);
}
}
}
Program 2
using System;
namespace SoloLearn {
class Program {
static void bubbleSort(int[] arr) {
bool swapped;
for(int i = 0; i < arr.Length – 1; i++) {
swapped = false;
for(int j = 0; j < arr.Length – i – 1; j++) { if(arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true;
}
}
if(swapped == false)
break;
}
}
static void printArray(int[] arr) {
for(int i = 0; i < arr.Length; i++) {
Console.Write(arr[i] + " ");
}
}
static void Main(string[] args) {
int [] arr = {5, 2, 42, 6, 1, 3, 2};
E(arr);
printArray(arr);
}
}
}
Code 3
using System;
public class BubbleSort
{
public static void Main()
{
// Array yang akan diurutkan
int[] intArray = new int[] { 1, 3, 7, 6, 5, 2, 10, 25, 4 };
// Menampilkan array sebelum diurutkan
Console.WriteLine("Array sebelum diurutkan:");
foreach (var item in intArray)
{
Console.Write("{0} ", item);
}
// Proses bubble sort
for (int i = 0; i < intArray.Length; i++)
{
for (int j = 0; j < intArray.Length - 1; j++)
{
if (intArray[j] > intArray[j + 1])
{
// Tukar posisi angka
int temp = intArray[j + 1];
intArray[j + 1] = intArray[j];
intArray[j] = temp;
}
}
}
// Menampilkan array setelah diurutkan
Console.WriteLine("\nArray setelah diurutkan:");
foreach (var item in intArray)
{
Console.Write("{0} ", item);
}
Console.WriteLine("\n");
}
}
/*
Hasil:
Array sebelum diurutkan:
1 3 7 6 5 2 10 25 4
Array setelah diurutkan:
1 2 3 4 5 6 7 10 25
*/
Program Bubble Sort pada Python
Code 1
import random
nums=[random.randint(0,20) for i in range(6)] print("List To Be Sorted -\n{}\n".format(nums))
run=True
while run:
good=True
for i in range(len(nums)-1):
if nums[i] > nums[i+1]:
x=nums[i+1] y=nums[i] nums[i]=x
nums[i+1]=y
good=False
print(nums)
if good == False:
continue
else:
run=False
print("\nSorted List -\n{}".format(nums))
Hasil :


Code 2
def bubbleSort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1] :
arr[j], arr[j+1] = arr[j+1], arr[j]
arr = [1,3,7,6,5,2,10,25,4]
bubbleSort(arr)
print ("Hasil dari Bubble Sort:")
for i in range(len(arr)):
print ("%d" %arr[i]),
Code 3
def bubbleSort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# traverse the array from 0 to n-i-1
# Swap if the element found is greater
# than the next element
if arr[j] > arr[j+1] :
arr[j], arr[j+1] = arr[j+1], arr[j]
# Driver code to test above
arr = [1,3,7,6,5,2,10,25,4]
bubbleSort(arr)
print ("Sorted array is:")
for i in range(len(arr)):
print ("%d" %arr[i]),
Program Bubble Sort pada Ruby
Code 1
def bubbleSort(arr)
swapped = true
for i in 0 … (arr.length – 1)
swapped = false
for j in 0 … (arr.length – i – 1)
if arr[j] > arr[j + 1] arr[j], arr[j + 1] = arr[j + 1], arr[j] swapped = true
end
end
break if swapped == false
end
end
nums = [3, 2, 1, 5, 21, 8, 6, 9, 4, 7] bubbleSort(nums)
p nums
Code 2
arr = [1,3,7,6,5,2,10,25,4]
def bubble_sort(array)
n = array.length
loop do
swapped = false
(n-1).times do |i|
if array[i] > array[i+1]
array[i], array[i+1] = array[i+1], array[i]
swapped = true
end
end
break if not swapped
end
array
end
p bubble_sort(arr)
# Hasil : [1, 2, 3, 4, 5, 6, 7, 10, 25]
Demikianlah postingan tentang Bubble Sort, semoga bisa bermanfaat buat kita semua